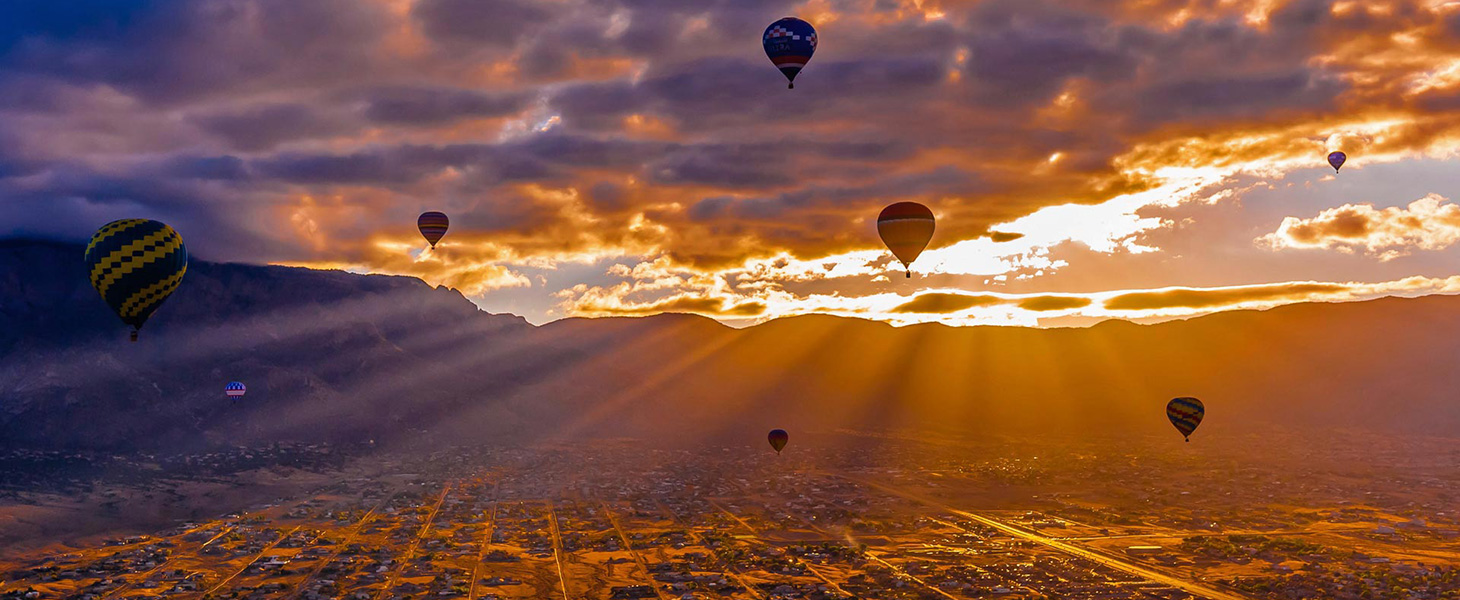
C++面向对象--类(Human类)
预备知识:
1.enum枚举
2.typedef enum 别名
3.vector 容器
4.指针
5.stringstream 流的输入输出
6.*与->的优先级 后者大于前者
7.?: 三目运算符的使用
8.引用的使用,局部变量的生存周期
添加头文件
#include<iostream> #include<string> #include<vector> //vector容器 #include<sstream>//istringstream、ostringstream 和 stringstream using namespace std;
Human.h
#pragma once #include<iostream> #include<string> #include<vector> #include<sstream> using namespace std; typedef enum gender { MAN, WOMAN }gender_t; class Human { public: Human(void); Human(const string&nick,int age,gender_t gender); virtual ~Human(void); string getNick()const; int getAge()const; gender_t getGender()const; Human* getLover()const; const vector<Human*> &getFriends()const;//需要返回引用,否则返回值为局部变量,函数块执行完毕,对象被销毁。外部使用的时候会出错 string describes(); void addFriend(Human &other); void delFriend(Human &other); void marry(Human&other); void divorce(); private: string nick;//昵称 int age; gender_t gender; vector<Human*> friends; Human *lover; };
Human.cpp
#include "Human.h" Human::Human(void) { this->lover = NULL; } Human::~Human(void) { } Human::Human(const string&nick,int age,gender_t gender) { this->nick = nick; this->age = age; this->gender = gender; this->lover = NULL; } string Human::getNick()const { return nick; } int Human::getAge()const { return age; } gender_t Human::getGender()const { return gender; } Human* Human::getLover()const { return lover; } const vector<Human*> & Human::getFriends()const { return friends; //返回引用类型,否则friends为复制的对象 函数结束就被销毁。 } string Human::describes() { stringstream rct; //流的输入输出 rct<<nick<<"-age:"<<age<<"-gender:"<<(gender==0?"男":"女"); //?: 三目运算符 return rct.str();//转换为string类型 } void Human::addFriend(Human &other) { friends.push_back(&other);//vector数值插入 } void Human::delFriend(Human &other) { vector<Human*>::iterator it; //C++11 可使用 auto自动匹配类型 for (it = friends.begin();it !=friends.end();) { if (*it == &other) //vector元素为指针 { it = friends.erase(it); }else { it++; } } } void Human::marry(Human&other) { lover = &other; other.lover = this; } void Human::divorce() { lover->lover = NULL; lover = NULL; }
main.cpp 测试
#include "Human.h" int main() { Human wbq("王宝强",30,MAN); Human mr("马蓉",28,WOMAN); Human hj("何炅",45,MAN); Human zxc("周星驰",60,MAN); cout<<wbq.describes()<<endl; wbq.marry(mr); cout<<wbq.getNick()<<"的配偶是:"; wbq.getLover()->describes(); cout<<wbq.getNick()<<"离婚"<<endl; wbq.divorce(); if (wbq.getLover() ==NULL) { cout<<wbq.getNick()<<"单身"<<endl; } wbq.addFriend(hj); wbq.addFriend(zxc); vector<Human*>::const_iterator it; it = wbq.getFriends().begin(); for (;it!=wbq.getFriends().end();) { cout<<(*it)->describes()<<endl; //(*it) 要加括号 ->的优先级 高于*的优先级 it++; } wbq.delFriend(hj); cout<<wbq.getNick()<<"与"<<hj.getNick()<<"断交"<<endl; for (it = wbq.getFriends().begin();it!=wbq.getFriends().end();it++) { cout<<(*it)->describes()<<endl; } system("pause"); return 0; }
运行结果:
王宝强-age:30-gender:男
王宝强的配偶是:王宝强离婚
王宝强单身
何炅-age:45-gender:男
周星驰-age:60-gender:男
王宝强与何炅断交
周星驰-age:60-gender:男
注意:getFriends() 函数一定要返回引用类型
const vector<Human*> & Human::getFriends()const
否则会崩溃:vector iterators incompatible
cout<<(*it)->describes()<<endl; //(*it) 要加括号 ->的优先级 高于*的优先级
留言评论
暂无留言