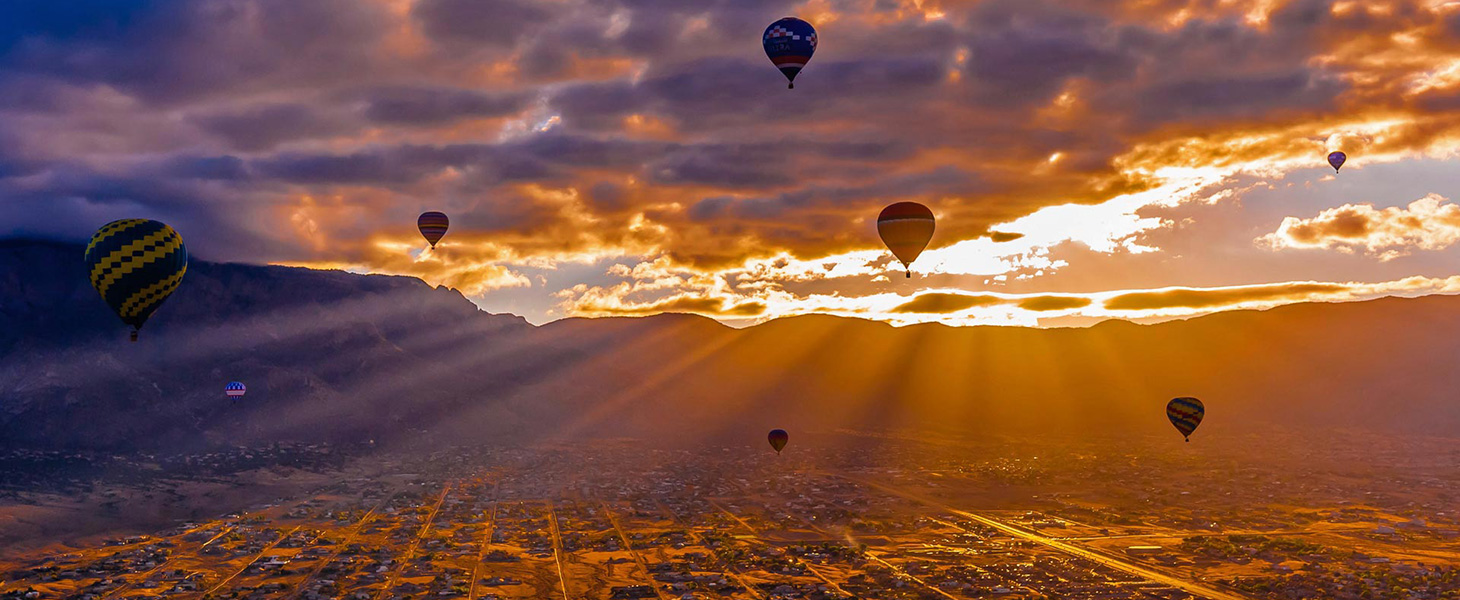
CriticalSection临界区Demo
头文件
#include<windows.h>
关键接口
CRITICAL_SECTION cs;
InitializeCriticalSection(&cs);
EnterCriticalSection(&cs);
LeaveCriticalSection(&cs);
DeleteCriticalSectrion(&cs);
Demo1:
#include <windows.h> #include <iostream> #include <thread> int main() { CRITICAL_SECTION cs; InitializeCriticalSection(&cs); int nCount = 0; std::thread th1([&] { int nCnt = 0; while (nCnt++ < 1000) { EnterCriticalSection(&cs); Sleep(5); nCount++; std::cout << "th1 nCount: " << nCount << std::endl; LeaveCriticalSection(&cs); } }); th1.detach(); std::thread th2([&] { int nCnt = 0; while (nCnt++ < 1000) { EnterCriticalSection(&cs); Sleep(5); nCount++; std::cout << "th2 nCount: " << nCount << std::endl; LeaveCriticalSection(&cs); } }); th2.detach(); system("pause"); return 0; }
Demo2:
#include <windows.h> #include <iostream> #include <thread> CRITICAL_SECTION cs; int nCount = 0; void thread_func1() { int nCnt = 0; while (nCnt++ < 1000) { EnterCriticalSection(&cs); Sleep(1); nCount++; std::cout << "th1 nCount: " << nCount << std::endl; LeaveCriticalSection(&cs); } } void thread_func2() { int nCnt = 0; while (nCnt++ < 1000) { EnterCriticalSection(&cs); Sleep(1); nCount++; std::cout << "th2 nCount: " << nCount << std::endl; LeaveCriticalSection(&cs); } } int main() { InitializeCriticalSection(&cs); std::thread th1(thread_func1); th1.detach(); std::thread th2(thread_func2); th2.detach(); system("pause"); return 0; }
留言评论
暂无留言