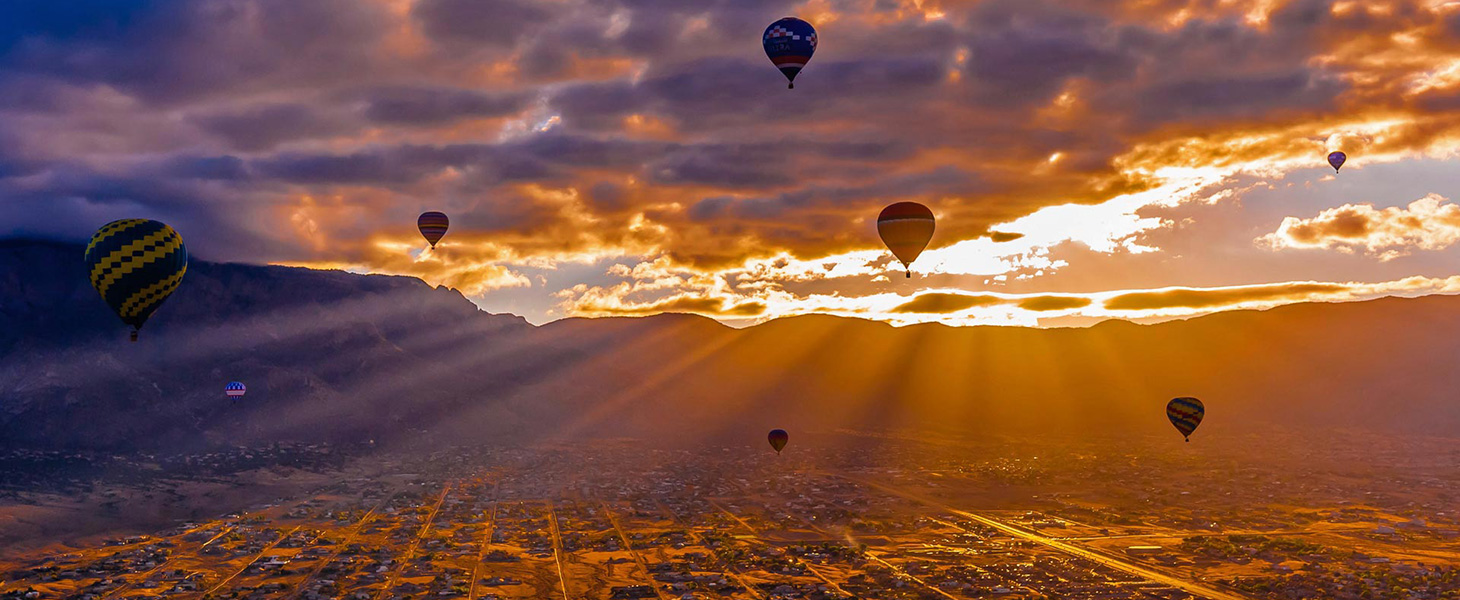
C++《数据结构与算法》-->顺序表
#include<iostream> using namespace std; #define MAX_SIZE 50 typedef struct { int *elems;//顺序表的首地址 int length;//顺序表的元素数量 int size;//顺序表的空间大小 }SqList; //初始化顺序表 bool initList(SqList & L){ L.elems = new int[MAX_SIZE];//申请堆空间分配内存 if(!L.elems){ cout<<"创建失败!"<<endl; return false; } L.length = 0; L.size = MAX_SIZE; cout<<"创建成功!"<<endl; return true; } //输出顺序表元素 void printList(SqList &L){ if(L.length==0){ cout<<"当前顺序表为空没有元素可以输出!"<<endl; } for(int i=0;i<L.length;i++){ cout<<"第"<<(i+1)<<"个元素:"<<L.elems[i]<<endl; } } //添加顺序表元素 bool addListElem(SqList &L, int e){ if(L.length >= L.size){ cout<<"顺序表已满!添加失败!"<<endl; return false; } L.elems[L.length] = e; L.length++; return true; } //指定位置插入顺序表元素 bool insterListElem(SqList &L, int i, int e){ if(L.length >= L.size){ cout<<"顺序表已满!插入失败!"<<endl; return false; } if(i<0 || i>L.size){ cout<<"插入位置错误!插入失败!"<<endl; return false; } for(int j = L.length-1; j>= i;j--){ L.elems[j+1] = L.elems[j]; } L.elems[i] = e; L.length++; return true; } //删除指定位置元素 bool deleteListElem(SqList &L, int i){ if(L.length <= 0){ cout<<"当前顺序表为空,没有可删除的元素!"<<endl; return false; } if(i< 0 || i> L.length){ cout<<"删除失败,要删除的位置不在有效范围内!"<<endl; return false; } if(i==L.length){ L.length--; return true; } for(int j = i;j <= L.length;j++){ L.elems[j-1] = L.elems[j]; //a cout<<" "<<L.elems[j-1]<<" "<<L.elems[j]; } L.length--; return true; } //销毁顺序表 释放堆上的内存 void destroyList(SqList &L){ if(L.elems) delete []L.elems; L.length = 0; L.size = 0; cout<<"销毁顺序表,释放堆内存。"<<endl; } int main(void){ SqList list; if(initList(list)){ cout<<"恭喜你顺序表创建成!"<<endl; } printList(list); cout<<"开始添自动加顺序表元素:"<<endl; for(int i = 0;i<5;i++){ if(!addListElem(list,i)){ cout<<"添加失败!"<<endl; break; } } cout<<"手动输入顺序表元素:"<<endl; int e; cin>>e; while(e!= 999){ if(!addListElem(list,e)){ cout<<"手动添加失败!"<<endl; break; } cin>>e; } printList(list); cout<<"插入顺序表元素:"<<endl; int index; cin>> index; cin>>e; while(e!= 999){ if(!insterListElem(list,index,e)){ break; } cin>>e; } printList(list); cout<<"删除末尾元素:"<<endl; deleteListElem(list,list.length); printList(list); cout<<"删除第二个位置的元素:"<<endl; deleteListElem(list,2); printList(list); //销毁顺序表 destroyList(list); return 0; }
运行结果:
留言评论
暂无留言